Cannot Pin ‘Torch.Cuda.Longtensor’ Only Dense CPU Tensors Can Be Pinned
Getting the “Cannot Pin ‘torch.cuda.LongTensor’ Only Dense CPU Tensors Can Be Pinned” error? GPU tensors can’t be pinned—convert them to CPU first using .cpu() to enable pinning and speed up data transfer.
In this guide, we’ll explain why this error occurs and give you practical solutions to resolve it. Whether you’re a beginner or an experienced PyTorch user, you’ll walk away with clear insights and effective fixes!
Understanding ‘Cannot Pin ‘torch.cuda.longtensor’ Only Dense CPU Tensors Can Be Pinned’
This error happens because only dense CPU tensors can be pinned, while CUDA tensors (GPU tensors) cannot.
Pinning memory transfers CPU-GPU data faster, but it’s a feature designed for CPU memory. Since CUDA tensors are already in GPU memory, there’s no need for pinning.
If you’re getting this error, you’re trying to pin a tensor that isn’t in the correct format. The fix is simple—move the tensor to the CPU before pinning. This ensures smooth deep-learning training and better performance.
1. What is Pinned Memory?
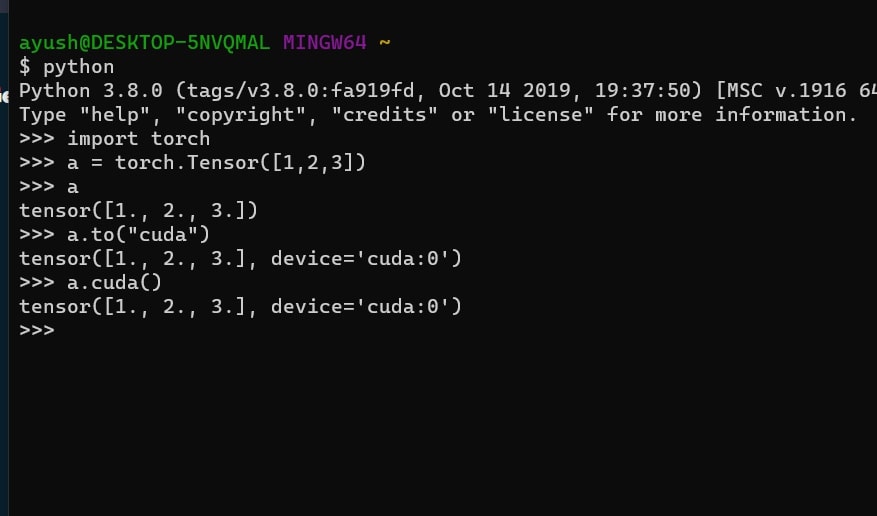
Pinned memory is a special CPU memory that helps speed up data transfers between CPU and GPU. Usually, data moves between them slowly because the CPU uses pageable memory, which can be swapped in and out.
But pinned memory stays fixed in place, making transfers much faster. This is why PyTorch uses pinned memory in its DataLoader—to speed up batch processing.
However, only CPU tensors can use pinned memory. If your tensor is on the GPU, move it to the CPU before pinning to avoid errors.
A. Restrictions on Pinning long Tensors on the GPU
Pinning memory only works for CPU tensors. It cannot be pinned if your tensor is on the GPU (torch.cuda.LongTensor). Here’s why:
- Pinning is a CPU feature—the GPU already has fast memory.
- CUDA tensors don’t need pinning since they’re already on the GPU.
- LongTensors are often sparse (not stored in a continuous block), which makes pinning difficult.
To avoid this issue, convert the tensor to CPU before pinning:
Tensor = tensor.cpu()
This simple step prevents errors and ensures smooth performance.
B. Handling the Error
If you see this error, don’t worry—it’s easy to fix! PyTorch is telling you that your tensor is not in the correct format for pinning. To fix it:
- Move the tensor to the CPU first:
tensor = tensor.cpu()
- Make sure it’s a dense tensor (not sparse):
If tensor.is_sparse:
tensor = tensor.to_dense()
- Use a FloatTensor instead of LongTensor:
tensor = tensor.float()
These steps ensure your tensor can be pinned properly for faster training and better performance.
2. Next Steps
To prevent this issue in the future, always double-check your tensors before pinning.
- Use only dense CPU tensors for pinning.
- Move GPU tensors to the CPU before pinning.
- If using LongTensors, consider switching to FloatTensors.
- Optimize batch loading by enabling pin_memory=True in DataLoader.
By following these simple steps, you’ll avoid frustrating errors, speed up your model training, and take advantage of PyTorch’s powerful features.
What Are Tensors in PyTorch?
Tensors are like advanced arrays that store numbers in multiple dimensions. They work like NumPy arrays but are faster and more flexible.
PyTorch uses tensors for deep and machine learning because they can quickly run on CPUs and GPUs. You can store, manipulate, and process large datasets efficiently with tensors.
They support various mathematical operations, making them perfect for training AI models. Whether working with images, text, or numbers, PyTorch tensors help you handle data smoothly for better performance.
1. Types of Tensors
PyTorch has different types of tensors for various tasks:
- Dense Tensors – Store all values in a continuous memory block (most common).
- Sparse Tensors – Store only non-zero values, saving memory for large datasets.
- FloatTensors – Used for floating-point numbers, ideal for deep learning.
- LongTensors – Store integer values, often used for indexing.
- Boolean Tensors – Hold True/False values for logical operations.
Each tensor type is optimized for different tasks, helping you improve speed and memory usage in PyTorch.
Role of Dense CPU Tensors in Pinning
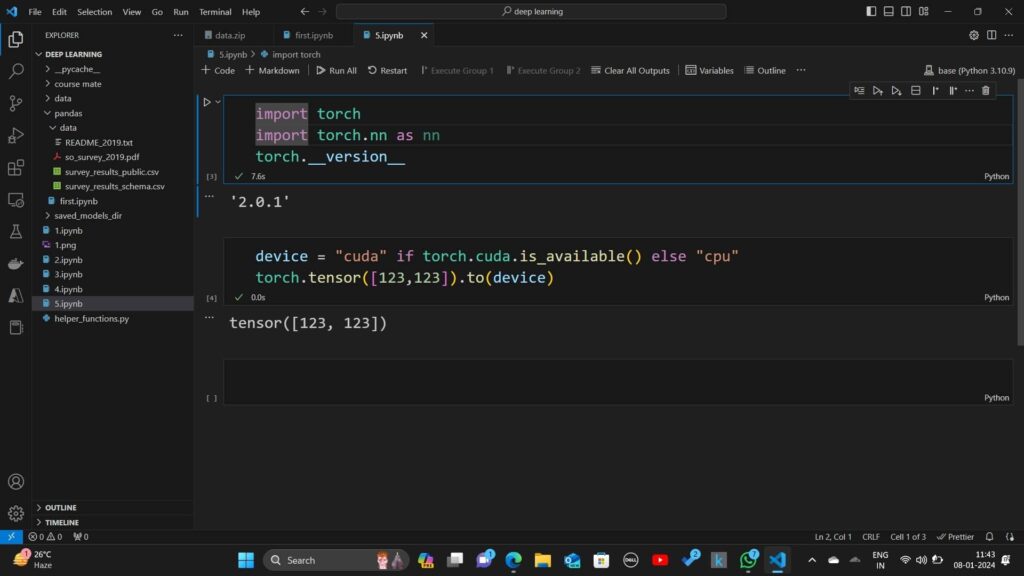
Dense CPU tensors are the only tensors that can be pinned in PyTorch. Pinning means locking a tensor in memory to speed up data transfer between the CPU and GPU.
Since dense tensors store data in a continuous memory block, they allow faster processing. Sparse or GPU tensors cannot be pinned because their memory is not constant. Pinning helps deep learning models run efficiently by reducing transfer delays.
What Are CUDA Tensors?
CUDA tensors are special tensors that run on the GPU, making computations much faster than CPU tensors. They are essential for deep learning because GPUs handle extensive calculations quickly.
Unlike CPU tensors, CUDA tensors don’t need pinning since they already live in GPU memory. To use CUDA tensors, move your data to the GPU using .to(‘cuda’). This improves performance, especially for AI and machine learning models.
Why Are LongTensors Non-Pinnable?
LongTensors store integer values, but they cannot be pinned in PyTorch. Pinning works best with floating-point tensors, which are commonly used in deep learning.
Since LongTensors often store categorical or index values, so they don’t need fast transfers as floating-point tensors. You’ll get an error if you try to pin a CUDA LongTensor. Convert it to a FloatTensor or work directly on the GPU to avoid issues.
Common Use Cases for Pinned Tensors
- Faster Data Transfer – Speeds up moving data between CPU and GPU.
- Efficient Data Loading – Helps PyTorch’s DataLoader load data quickly.
- Better GPU Utilization – Reduces waiting time during training.
- Handling Large Datasets – Useful for deep learning models with massive data.
- Optimizing Batch Processing – Makes processing multiple samples smoother.
- Reducing Latency – Improves real-time AI performance.
- Enhancing Training Speed – Helps models train faster by avoiding memory bottlenecks.
- Improving Data Preprocessing – Allows efficient handling of input data before training.
Troubleshooting Similar Issues
1. Check If the Tensor Is on the CPU
Only CPU tensors can be pinned in PyTorch. Use tensor.device to check its location. If it’s on the GPU, move it to the CPU using .to(‘cpu’). This simple fix prevents pinning errors and improves data transfer efficiency.
2. Ensure the Tensor Is Dense
Sparse tensors cannot be pinned because they don’t store data in a continuous block. If you’re working with a sparse tensor, convert it to a dense format using .to_dense(). This allows pinning and speeds up training.
3. Verify the Tensor Data Type
Long tensors cannot be pinned. If you’re using one, convert it to a Float Tensor using .float(). Floating-point tensors are better for deep learning and work with pinned memory, improving performance and reducing errors.
4. Check If the Tensor Is Contiguous
Non-contiguous tensors may cause unexpected pinning errors. Ensure your tensor is stored contiguously in memory using .contiguous(). This step helps PyTorch process data efficiently and prevents issues when transferring tensors between CPU and GPU.
5. Debug with Smaller Tensors
If pinning fails, start with a small tensor to check if the issue is with memory size or tensor properties. If a small tensor works, gradually increase the size. This method helps pinpoint the exact problem and speeds up debugging.
Dataloader Pin Memory
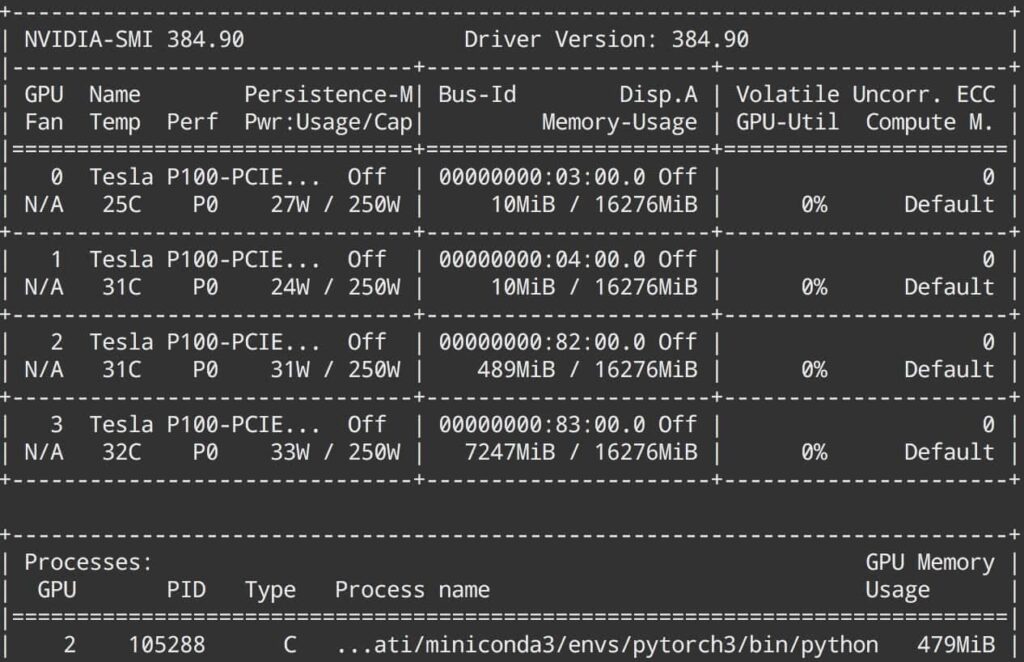
The pin_memory=True option in PyTorch’s DataLoader speeds up data transfer between CPU and GPU. When enabled, it locks tensors in memory, making GPU access faster.
This is useful for large datasets in deep learning. However, it only works with CPU tensors, so ensure your data is correctly stored before enabling it.
Pytorch Pin Memory Slow
Sometimes, pin_memory=True can slow down performance instead of improving it. This happens if too many pinned tensors overload system memory or your CPU is outdated.
Try reducing batch size, upgrading hardware, or disabling pinning to see if performance improves. Testing different settings helps find the best configuration for your system.
Runtimeerror: Caught Runtimeerror In Pin Memory Thread For Device 0
This error usually happens due to low memory, incorrect tensor formats, or PyTorch bugs. Restarting your training script can help.
If the problem continues, try disabling pin_memory, checking your DataLoader settings, or updating PyTorch. Reducing num_workers can also prevent crashes in the pin memory thread.
Runtimeerror: Pin Memory Thread Exited Unexpectedly
This means the pin memory thread stopped working suddenly, often due to system memory issues or PyTorch errors.
Fix it by reducing batch size, disabling pin_memory, or restarting your system. If using multiple workers, try lowering num_workers. Keeping PyTorch updated helps prevent such errors.
Using Pin_memory=False As Wsl Is Detected This May Slow Down The Performance
If you’re using Windows Subsystem for Linux (WSL), PyTorch may disable pin_memory because WSL doesn’t handle pinned memory well.
This can slow training down. To fix it, consider switching to WSL 2 with GPU support or using a native Linux system for better performance.
FAQs
1. What is the difference between torch Tensor and torch CUDA Tensor?
A torch.Tensor is stored in CPU memory, while a torch.cuda.Tensor is stored in GPU memory for faster computations using CUDA. CUDA tensors help speed up deep learning tasks.
2. What is pinned memory in PyTorch?
Pinned memory locks CPU tensors in RAM, making data transfers between CPU and GPU much faster. This improves performance in deep learning models by reducing transfer delays.
3. What is the difference between CPU and GPU Tensor?
CPU tensors run on the processor, while GPU tensors use CUDA for faster parallel computations, making them better for deep learning and other intensive tasks.
4. What is the default Dtype of torch Tensor?
The default dtype of a torch.Tensor is torch.float32, which balances accuracy and memory usage well, making it ideal for machine learning tasks.
5. Is Torch faster than TensorFlow?
PyTorch is often faster for research and dynamic models, while TensorFlow is better for large-scale deployment and production due to its optimizations.
6. What is the difference between CUDA Cores and Tensor Cores?
CUDA Cores handle general GPU tasks, while Tensor Cores accelerate deep learning operations, like matrix multiplications, improving AI and machine learning performance.
7. Does PyTorch support Tensor Cores?
Yes! PyTorch automatically uses Tensor Cores for faster deep learning computations, improving efficiency when working with mixed-precision training on supported GPUs.
8. What is the difference between detach and CPU in PyTorch?
.detach() removes a tensor from the computation graph, while .cpu() moves a tensor from GPU to CPU memory for further processing.
9. Can I pin other types of GPU Tensors to CPU memory?
No, only CPU tensors can be pinned. First, to pin a GPU tensor, convert it to a CPU tensor using .cpu().
10. Are there any alternatives to pinning ‘torch.cuda.LongTensor’?
Yes! You can convert it to a torch.FloatTensor or use efficient data transfer methods like asynchronous memory copy for faster performance.
Conclusion
Understanding why “Cannot Pin ‘torch.cuda.LongTensor’ Only Dense CPU Tensors Can Be Pinned” helps you fix it quickly. Always convert GPU tensors to CPU before pinning. Following best practices ensures smooth training, faster data transfers, and better PyTorch performance!